This article focuses on “How to implement In-Memory Caching in ASP.NET Core?”
What is Caching?
Caching is a method to deliver data faster to the client so that information can be served a lot quicker for any future requests. So, we take the most often utilized information and duplicate it into brief capacity so it very well may be gotten to a lot quicker in later calls from the client.
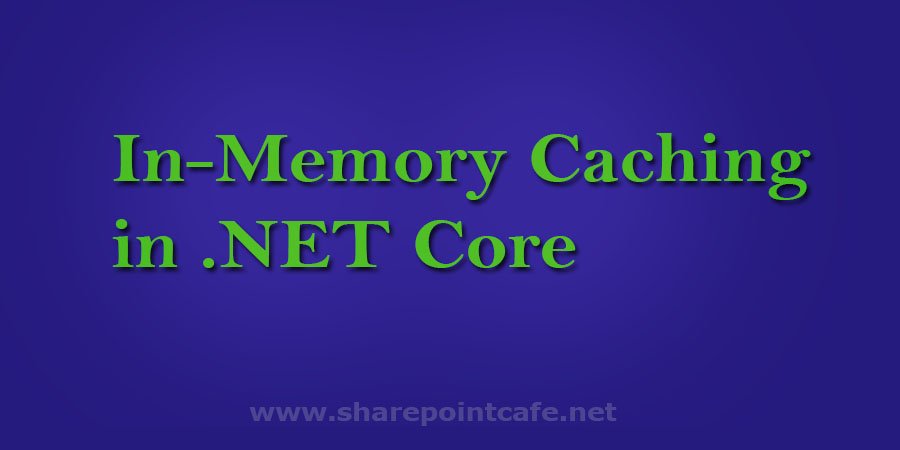
In-Memory Caching
In this article, we will explore in-memory caching and will see how to implement this.
An in-memory cache is stored in the memory of a single application server. This is the most straightforward approach to enhancing the application performance. The principal benefit of In-memory caching is it is faster and it’s reasonable for small scale applications.
In-Memory Caching implementation in .NET 5
Open Visual Studio 2019 or later and create a .NET based API.
Open Startup.cs file and add below line in ConfigureServices() method.
services.AddMemoryCache();
Open your Controller class and add this namespace
using Microsoft.Extensions.Caching.Memory;
Declare this variable
private readonly IMemoryCache _inMemoryCache;
Write below the action method
[HttpGet]
public async Task<IActionResult> GetProductList()
{
var cacheKey = "productList";
if (!_inMemoryCache.TryGetValue(cacheKey, out List<Products> productList))
{
productList = await _context.Products.ToListAsync();
//setting up cache options
var cacheExpiryOptions = new MemoryCacheEntryOptions
{
AbsoluteExpiration = DateTime.Now.AddSeconds(50),
Priority = CacheItemPriority.High,
SlidingExpiration = TimeSpan.FromSeconds(20)
};
_inMemoryCache.Set(cacheKey, productList, cacheExpiryOptions);
}
return Ok(productList);
}
Open the Postman tool and check the response time.
Before caching implementation, it took 1789 ms

Post Caching implementation, it took 44 ms. Is not that a drastic performance improvement?
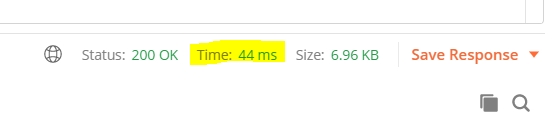
Absolute Expiration and Sliding Expiration
You may notice that in the Cache Expiry options, we have Absolute Expiry time and Sliding Expiry time.
Absolute Cache Expiry means it will expire the cache after a defined time doesn’t matter whether it has been used or not.
On another side, Sliding Cache expiry means it will expire the cache if and only if it has not been accessed during the defined time.
Where to apply In-Memory Caching in ASP.NET Core?
Now you may plan to implement In-Memory Caching in ASP.NET Core and the question is when and where to use this caching mechanism. In case you have common data to present to all users of the application. In this scenario, we can implement in-memory caching.
Takeaway
Caching is a mechanism to improve the application performance drastically. In-memory caching in ASP.NET Core is the fastest way to deliver data to clients for small scale applications.
I hope you like this article.
Read our top articles –
- How to implement Dependency Injection in .NET 5?
- .NET 5 Web API CRUD with Angular and Entity Framework
- In-Process and Out-Of-Process hosting models in ASP.NET Core
- How to migrate from ASP.NET Core 3.1 to .NET 6.0?