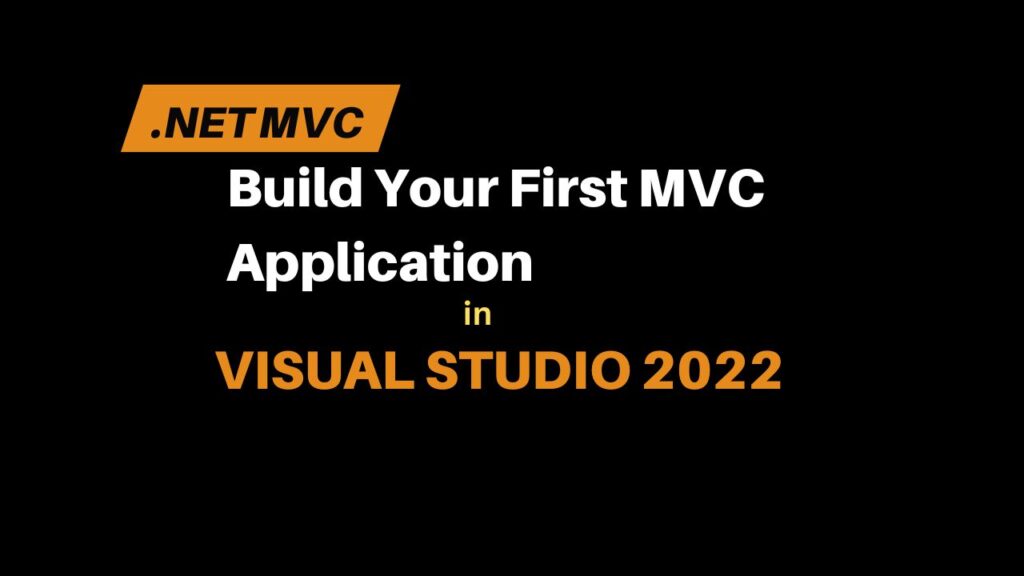
In this article, we will demonstrate How to build the first MVC application in Visual Studio 2022 using .NET 6.
Also, we will see how the .NET 6-based MVC project is different from than .NET Core-based MVC project.
Prerequisites
- .NET 6 (What’s new in .NET 6?)
- Visual Studio 2022 (How to install Visual Studio 2022?)
Create MVC application in Visual Studio 2022
Follow the below steps to create your first MVC application in Visual Studio 2022.
- Open Visual Studio 2022 and Click on Create a new project.
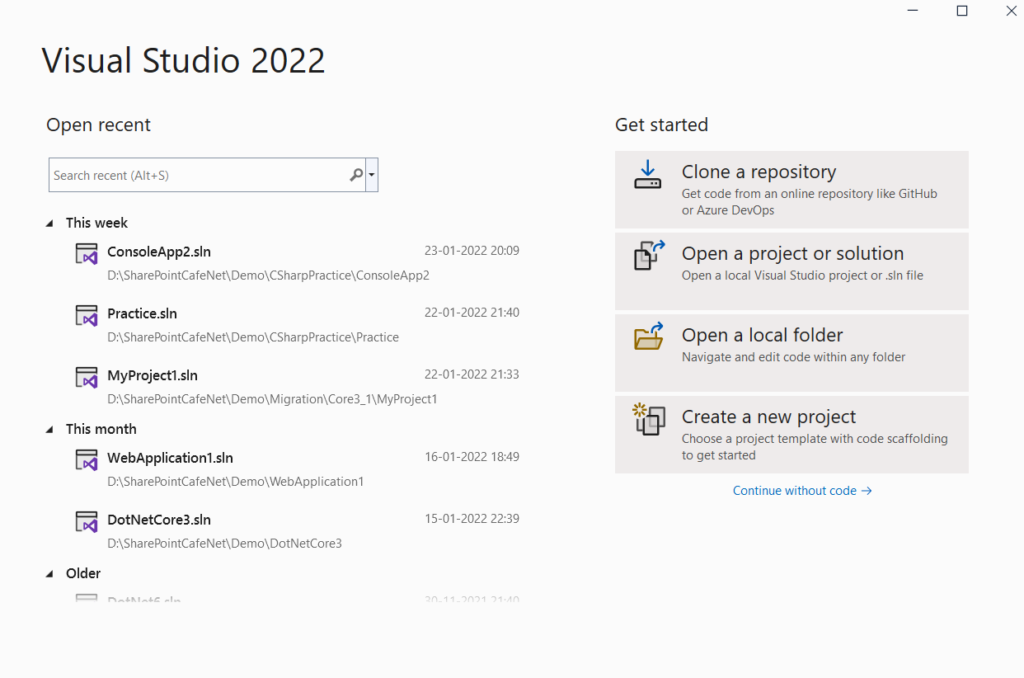
2. Search for MVC and select ASP.NET Core Web App(Model-View-Controller) as shown in the below image.
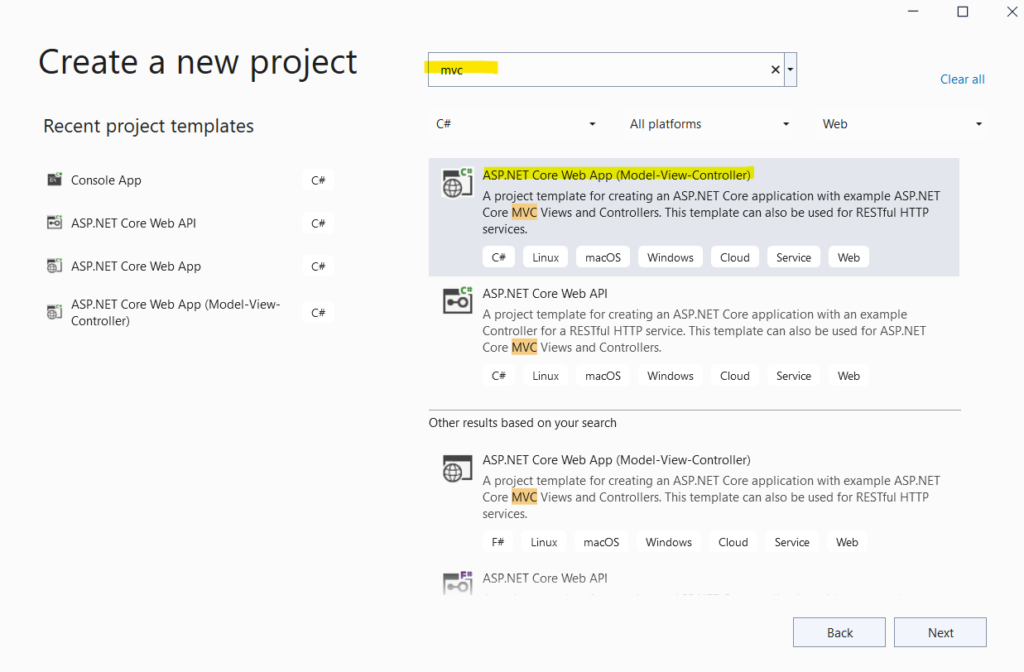
3. Give a name to the project and select Location.
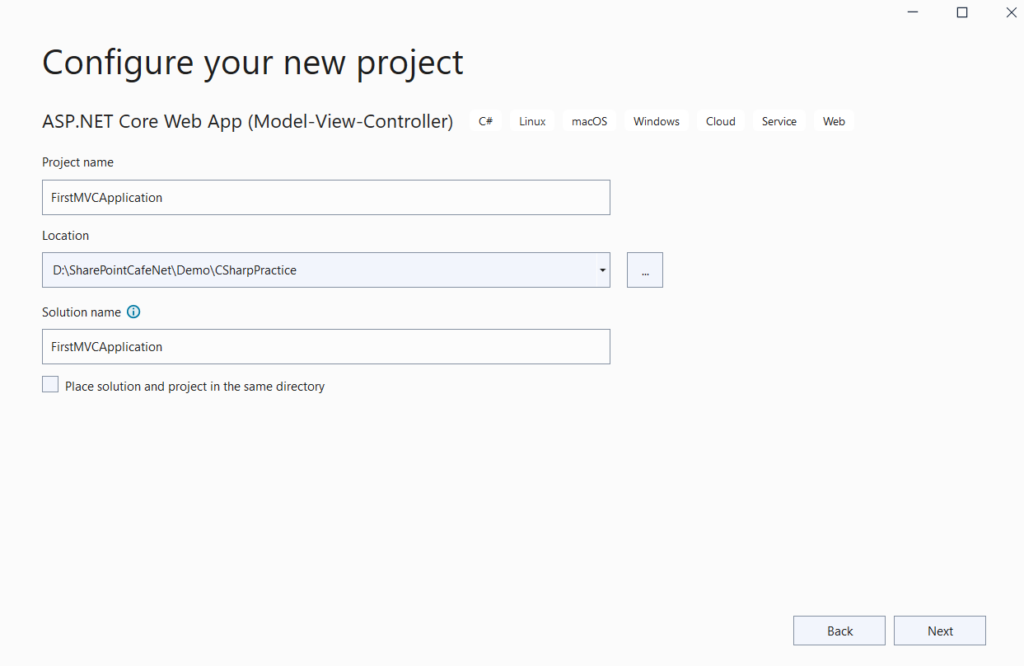
4. Select Framework as .NET 6, leave other selections as default and click on Create.
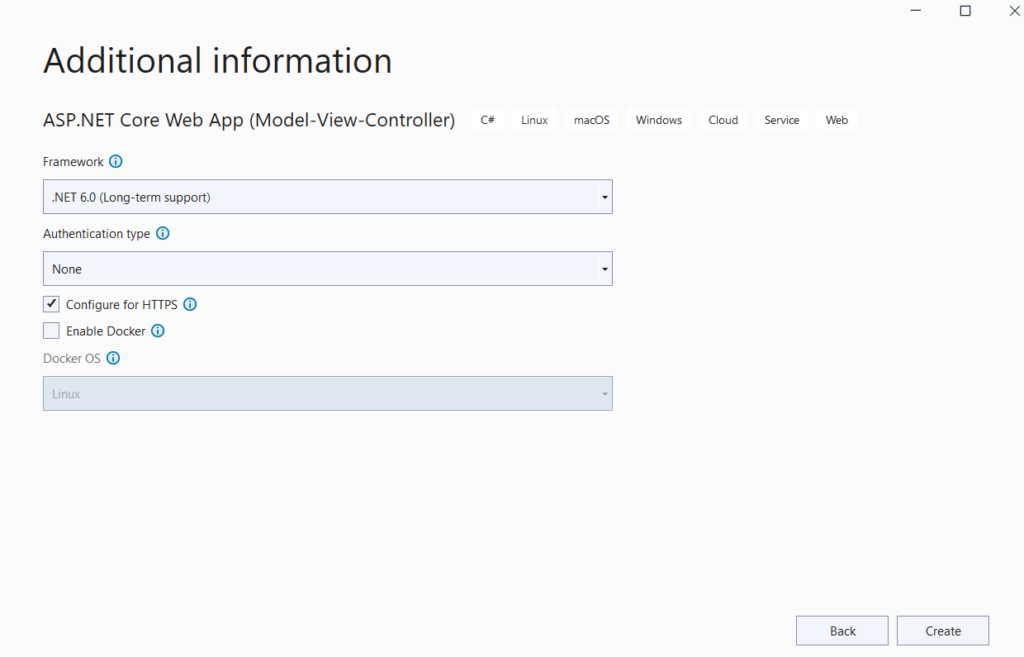
Below is the directory structure of the .NET 6 MVC application.
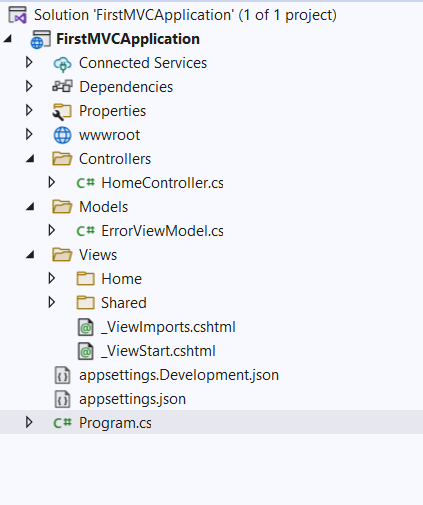
MVC Application Code Explanation
Below is the code snippet of the HomeController.cs
file.
There is no change here if we talk about code structure, constructor, and action methods. It looks similar to the .NET Core 3.1 Controller class.
using FirstMVCApplication.Models;
using Microsoft.AspNetCore.Mvc;
using System.Diagnostics;
namespace FirstMVCApplication.Controllers
{
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger)
{
_logger = logger;
}
public IActionResult Index()
{
return View();
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
Program.cs File – This is one of the major changes in .NET 6. In earlier versions of .NET Core like 3.1 Program.cs file contains 2 major methods –
One is Main() and the next one is CreateHostBuilder()
Now in .NET 6, you may notice that CreateBuilder()
method is the very first line in Program.cs
file :
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllersWithViews();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
If you have worked in earlier versions of ASP.NET Core then you have noticed the Startup.cs
file but here is the twist, there are no more startup.cs
file in Dot NET 6 version. It can be thought of as a major change in the .NET 6 framework.
.NET 6 startup.cs
file code is merged in the Program.cs file
.
For reference, look at the startup.cs
file below which is part of MVC .NET Core 3.1
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.HttpsPolicy;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace MVC3_1
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
}
}
So, by looking at .NET 6 Program.cs
file and .NET Core 3.1 startup.cs
file we can easily identify that services.AddControllersWithViews();
was part of ConfigureServices()
method in Core 3.1 but it is part of the Program.cs
file in Dot NET 6.
Similarly, code snippets include built-in middleware in the startup.cs file is now part of the Program.cs
file in Dot NET 6.
Let’s run our first MVC application. Press F5 to see the output.
Summary –
So, in this way, we can build the first MVC application in Visual Studio 2022 and .NET 6. In general, we have noticed one significant change which is the startup.cs
file code snippets are now part of the Program.cs
file.
I hope you like this article.
Please share this blog within your tech group and in case you have any feedback, feel free to write it down in the comment box below.
You may read articles on AZ 900.