In this blog, we will see how to host an ASP.Net Core application on IIS server. Hosting ASP.Net Core app on IIS is not so different than hosting a traditional ASP.Net web application. If you are new to ASP.Net core, you may read this blog – ASP.Net Core Blog
Pre-requisite to host ASP.Net Core application on IIS
To demonstrate this blog I have below platform and software with me.
OS- Windows 10
Web Server – IIS
IDE – Visual Studio 2017
and .Net Core
Create Application in Visual Studio 2017
First, you need to create an ASP.Net Core application in Visual Studio 2017.
Open your Visual Studio.
File->New Project.
Select ASP.Net Core Web Application then select Web Application from the next dialog box.
Web Application in ASP.Net Core is a project template for creating an ASP.Net Core application with ASP.Net Core Razor pages.
You may see folders and files in solution explorer with name wwwroot and pages.
wwwroot contains static files for eg images, stylesheets, script files
Our Popular Blog – |
Pages folder contains Razor View pages which have .cshtml extension.
Press F5 to see the output.
Next, we will host and run the same application from IIS.
Hosting Model
There are 2 hosting models available for ASP.Net Core application.
Out of process model
An out-of-process model can be used with .Net Core V2.2 and earlier.
In this hosting model, a request hit IIS and then forwarded to ASP.Net Core application running Kestrel Web server.
The out-of-process model uses IIS as a proxy to forward the incoming requests to dotnet.exe.
In-Process model
In this hosting model, ASP.Net Core app runs in the same process as its worker process in IIS. The in-process hosting model is better than the Out-of-process hosting model. It is available for .Net core V2.2 and later.
The in-process hosting model uses IISHttpServer instead of Kestrel. IISHttpServer is hosted directly inside an application pool.
We can switch from the Out-of-process hosting model to the In-process hosting model easily.
A newer version of ASP.Net Core application automatically configures InProcess hosting, in case you are migrating from an older version then you have to do a project-level configuration.
Enable IIS on Windows 10
Check if IIS is installed or not on your machine. To do so, run inetmgr in Run window. If it runs then it is ok, else you have to add IIS from Windows feature in Control Panel – Add Remove Programs ->Turn On/Off Windows features.
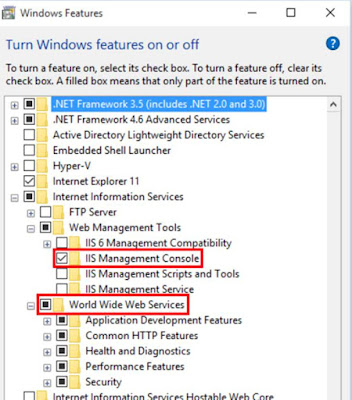
.Net Core Hosting Bundle
Once IIS is added to your machine, still you can not run your .Net Core application on IIS.
This is because you need to install .Net Core hosting bundle for IIS.
This bundle will install ASP.Net Core module for IIS.
You may download the same from the below link –
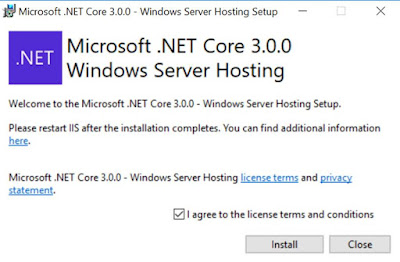
After installation is completed, either you restart the system or run below 2 commands from Visual Studio Command Prompt. (Run command prompt window with Admin rights)
1. net stop was /y
2. net start w3svc
The above 2 commands allow IIS to picks up the related changes.
Enable IIS Integration with .Net Core App
In program.cs file, CreateWebHostBuilder is being called for setting up a host that enables IIS integration.
public static IWebHostBuilder CreateWebHostBuilder(string[] args) => WebHost.CreateDefaultBuilder(args) .UseStartup<Startup>(); |
Deploy and Host Application on IIS
Now you are ready to host your .Net Core application on IIS.
First, publish your code in a local folder. While publishing the code Visual Studio will create web.config file in that folder.
In IIS Manager, open the server’s node in the Connections panel. Right-click the Sites folder. Select Add Website from the contextual menu.
Go to your application pool and edit it. Select No Managed Code from .NET CLR version.
Select your site from the left panel in IIS and double click on Modules from the middle panel.
In the Name column, check what ASP.Net Core module is showing AspNetCoreModule
or AspNetCoreModuleV2
Now, check your web.config
from the published folder. Make sure modules name in web.config
is the same as shown in the IIS module if it is same then leave it if it is not same then make changes in web.config
file.
In my case, it is AspNetCoreModuleV2 so I make changes in web.config
file accordingly.
<handlers> <add name=“aspNetCore“ path=“*“ verb=“*“ modules=“AspNetCoreModuleV2“ resourceType=“Unspecified“ /> </handlers> |
Now your ASP.Net Core application should run.
What is ASPNetCoreModule?
AspNetCoreModule is responsible to handle all incoming requests to IIS and manage those requests to route to ASP.Net Core application.
Idle Timeout Issue
If you are using the in-process hosting model, you may get an error that the application pool is getting idle.
To avoid app pool idle state, set the app pool’s idle timeout using IIS Manager:
Select Application Pools in the Connections panel.
Right-click the app’s app pool in the list and select Advanced Settings.
The default Idle Time-out (minutes) is 20 minutes. Set the Idle Time-out (minutes) to 0 (zero). Select OK.
Recycle the worker process.
If you like this blog, share this on social media.
Previous Blog – Introduction to .Net Core 2.0
Next Blog – Razor Pages vs MVC in ASP.Net Core
You may like other blogs –
Interview Questions and Answers Series –
MVC Interview Questions and Answers
Web API interview questions and answers