In this blog, we will see ASP.Net Core Razor Pages handler methods in detail. Also, we will explore how handler methods are invoked in Razor pages based on user action.
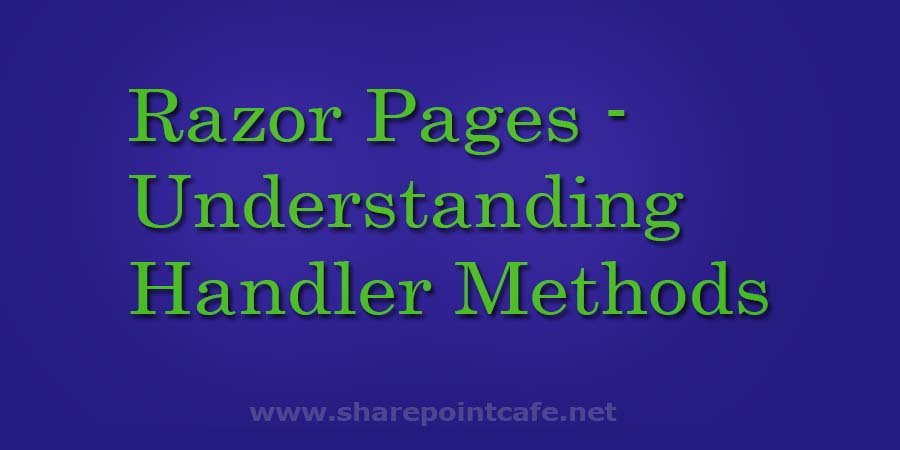
What are handler methods in .Net Core Razor pages?
Handler methods in ASP.Net Core Razor pages are available with nice features that will be loved by most developers.
Razor Pages handlers help to connect a user request to the methods written in the .cshtml.cs file.
Handler methods in Razor Pages are based on naming conventions.
In Razor pages, the basic methods work by matching HTTP verbs and they are prefixed with the word “On”.
Razor pages have naming conventions.
- OnGet()
- OnPost()
- OnGetAsync()
- OnPostAsync()
You may notice that all start with On, followed by either Get or Post and then followed by Handler Name which is optional.
Get Method
By default, OnGet() methods get added when a Razor page is created.
public void OnGet()
{
}
OnGet() method executes as soon as the Razor page loads in the browser.
POST Method
To add a POST method on Razor pages, add the below line in .cshtml file –
<form method="post"><button type="submit" class="btn btn-default">Submit</button></form>
And below code in .cshtml.cs file –
public void OnPost()
{
}
Once you click on the submit button, OnPost() method gets executed.
Multiple POST Handlers on a single Razor Page
Suppose, you have more than one form on a single Razor page. In this case how you will handle button events?
To handle this in Razor pages, we can use an asp-page-handler attribute with the form tag.
Let’s understand this with an example.
Append the asp-page-handler attribute value with OnPost or OnGet in .cs file.
Write the below code in .cshtml file –
<form asp-page-handler="save" method="post"><button type="submit" class="btn btn-default">Save Data</button></form>
<form asp-page-handler="edit" method="post"><button type="submit" class="btn btn-default">Edit</button></form>
<form asp-page-handler="delete" method="post"><button type="submit" class="btn btn-default">Delete Record</button></form>
You may notice that all form tag has a method-type post, but the asp-page-handler is different.
Write the below code in the .cshtml.cs file and append the asp-page-handler attribute value with the method name after OnPost.
public void OnPostSave()
{
//Write your logic for POST
}
public void OnPostDelete()
{
//Write your logic for POST
}
public void OnPostEdit()
{
//Write your logic for POST
}
How to pass Parameters in Razor Pages?
There are 2 ways to pass parameters to Razor page handlers.
1. Form Inputs
In Razor pages, I have designed a basic form to read the user input.
.cshtml file –
<form asp-page-handler="save" method="post">
<div class="form-group">
<label for="name">Product Name:</label>
<input type="text" name="name" class="form-control" id="name">
</div>
<div class="form-group">
<label for="desc">Product Description:</label>
<input type="text" name="description" class="form-control" id="description">
</div>
<div class="form-group">
<label for="cost">Product Cost:</label>
<input type="text" name="cost" class="form-control" id="cost">
</div>
<div class="form-group">
<label for="stock">Product Stock:</label>
<input type="text" name="stock" class="form-control" id="stock">
</div>
<button type="submit" class="btn btn-default">Save Data</button>
</form>
HTML Output –
.cshtml.cs code-
You may notice that data entered in the form can be read in the Post method parameter.

2. asp-route Tag helper
This is a similar process we have been using in traditional ASPX pages, but in Razor pages, it is quite different.
Another way to read parameters in Razor pages is by using an asp-route attribute with the form tag.
Below is the Cshtml code, as you can see asp-route-id is added with the form tag.
<form asp-page-handler=”edit” asp-route-id=”2″ method=”post”><button type=”submit” class=”btn btn-default”>Edit</button></form> |
Once the user clicks on the button it redirects to the below URL with an id parameter.
Summary:
Handler methods in Razor pages provide a clear structure and easy-to-understand naming convention. Although Razor pages have a few different attributes to handle Get and Post methods, the handler methods structure in Razor pages looks similar to MVC. So in this way, Razor pages provide a clear and clean structure of methods that the user is going to perform.
Hope this blog is helpful to you. Share this blog on social media.
Previous Blog – Razor pages vs MVC application in .Net Core
Next Blog – Database connection string in .Net Core
You may like other blogs –
- MVC Tutorial
- Web API Tutorial
- Angular Tutorial
- Learn TypeScript
- CRUD Example in Angular 7
- Razor Pages vs MVC in .Net Core
- Authentication and Authorization in MVC
Interview Questions and Answers Series –
MVC Interview Questions and Answers
Web API interview questions and answers