In this article, we will see about Filters in .NET MVC. We will learn what are filters in MVC and where can we use them in our application.
So let’s start and learn .NET MVC filters.
Previous Chapter: Data Validation and Annotation in .NET MVC
What are Filters in .NET MVC?
Suppose you have to execute some code before and after an action method in an MVC application. So how to overcome this situation? MVC filters are used to deal with this situation.
As we know in the MVC application a request first reaches to controller class and then goes to an action method.
However, in some cases, we may be required to execute our custom logic before and after executing action methods. We can implement this feature in MVC using
filters.
Filters in MVC is a custom class where we can write our logic to execute before or after action methods. We can apply filters to action methods or controller classes using an attribute.
Types of Filters in .NET MVC
.NET MVC offers 4 types of filters.
1. Authorization Filters
2. Action Filters
3. Result Filters
4. Exception Filters
1. Authorization Filters
The Filter executes before an action method to check the authenticity of a user who is going to execute the specific action method. Authorization filters get executed before any other filter. It means the Authorization filter is the first filter to execute.
Whenever a request comes from the browser, IAuthorizationFilter
. The onAuthorization
method executes and then goes to ActionFilter.
The authorization filter in MVC implements the IAuthorizationFilter
interface
Create a folder in solution and name it Custom.
Add a new class within this folder. Class – AuthFilterAttribute.cs
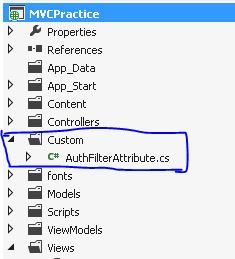
IExceptionFilter
has one method – OnAuthorization
public class AuthFilterAttribute:FilterAttribute, IAuthorizationFilter
{
public void OnAuthorization(AuthorizationContext filterContext)
{
filterContext.Controller.ViewBag.Message = “Authorization Filter Executed”;
}
}
The AuthFilterAttribute
class inherits the FilterAttribute
class and IAuthorizationFilter
interface, finally, we are implementing the OnAuhtorization
method.
We will add AuthFilter
on Index() method.
[AuthFilter]
public ActionResult Index()
{
return View();
}
<h2>@ViewBag.Message</h2>
2. Action Filter
Action filters in MVC implement IActionFilter
interface
IActionFilter – The OnActionExecuting
method executes before an action method gets executed and once the action method is executed IActionFilter.OnActionExecuted method gets invoked. Then request goes to the Result Filter.
This class inherits –
1. FilterAttribute class
2. IActionFilter interface
We can apply an action filter attributes either on an action method or a controller class. In case we apply the action filter attribute to a controller class then it will apply this to each action method within the controller class.
IExceptionFilter
has 2 methods – OnActionExecuting
and OnActionExecuted
public class CustomActionFilterAttribute : FilterAttribute, IActionFilter
{
public void OnActionExecuting(ActionExecutingContext filterContext)
{
filterContext.Controller.ViewBag.Message = “OnActionExecuting Begins”;
}
public void OnActionExecuted(ActionExecutedContext filterContext)
{
filterContext.Controller.ViewBag.Message = “OnActionExecuting Completed and OnActionExecuted Started”;
}
}
Built-In Action Filter
.NET MVC provides a built-in action filter as well. Each built-in action filters have its own specific tasks.
The OutputCache is one of the built-in action filter attributes. The purpose of the OutputCache
action filter is to cache the output.
We can apply OutputCache
with duration. The following code snippet will cache the output for 60 seconds.
[OutputCache(Duration=60)]
public ActionResult Index()
{
return View();
}
3. Result Filters
Result Filters in .NET MVC get executed before and after the result of an action method.
This filter in MVC implements the IResultFilter
interface including two methods – OnResultExecuting and OnResultExecuted.
To create a custom Result Filter, we need to implement the IResultFilter interface.
To implement custom logic before generating the result we must implement the OnResultExecuting method. In a similar way, to implement custom logic after generating the result we must implement the OnResultExecuted method.
public class CustomResultFilter:FilterAttribute, IResultFilter
{
public void OnResultExecuting(ResultExecutingContext filterContext)
{
filterContext.Controller.ViewBag.Message2 = “OnResultExecuting”;
}
public void OnResultExecuted(ResultExecutedContext filterContext)
{
filterContext.Controller.ViewBag.Message2 = “OnResultExecuted and Done”;
}
}
Here, This CustomResultFilter
class inherits-
1. FilterAttribute class
2. IResultFilter interface
4. Exception Filters
This filter executes when an action throws an exception in between any stage of execution in the pipeline.
Exception filter in .NET MVC inherits –
1. FilterAttribute class
2. IExceptionFilter interface
IExceptionFilter has one method – OnException
public class CustomExceptionFilter:FilterAttribute, IExceptionFilter
{
public void OnException(ExceptionContext filterContext)
{
filterContext.Controller.ViewBag.Message3 = “Exception Occured”;
}
}
You can apply these filters as an attribute on your Action method in the Controller class –
public class Default1Controller : Controller
{
// GET: /Default1/
[CustomActionFilter]
[AuthFilter]
[CustomResultFilter]
public ActionResult Index()
{
return View();
}
}
In the above sample code, I have stored the message in ViewBag and Printed it on View. Instead of storing a message in ViewBag, we can apply some logic that will execute.
<h2>@ViewBag.Message</h2>
<h2>@ViewBag.Message1</h2>
<h2>@ViewBag.Message2</h2>
<h2>@ViewBag.Message3</h2>
HandleError
is the built-in exception filter in .NET MVC.
FilterAttribute class inherits the Attribute class and IMvcFilter interface
Hope you understand the concept of MVC filters. If this article is useful to you then please share this blog within your community.
Keep Following – SharePointCafe.NET