A lambda expression is a short way to describe an anonymous function in C#. The abbreviated syntax of Lambda Expression helps to create Delegates and expressions. So, in this article, we will see Lambda Expression in C# with examples.
We can use the lambda function to quickly define a method and then pass it as an argument to another method that needs an expression or delegate.
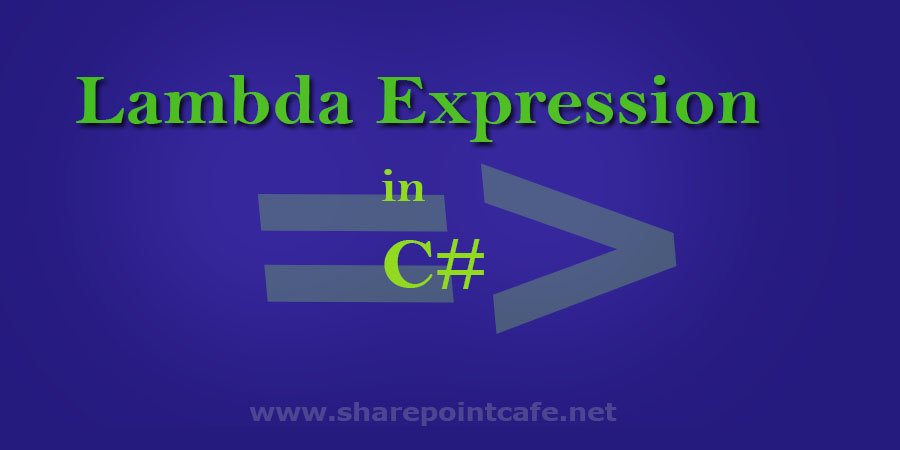
When querying data from databases or collections, LINQ (Language Integrated Query) is frequently used with lambda functions. Additionally, they are frequently utilized in asynchronous programming, functional programming in C#, and event handling.
Benefits of Using Lambda Expressions in C#
By utilizing lambda expressions in C#, you can support powerful language features like LINQ and functional programming while also making your code more readable, adaptable, and concise.
There are several benefits of using lambda expressions in C#, including:
- Conciseness: Lambda expressions allow you to write shorter and more readable code, by removing the need to define a separate named method for simple operations.
- Flexibility: Lambda expressions can be used wherever a delegate or an expression is expected, providing a flexible way to define functionality on the fly.
- LINQ support: Lambda expressions are a crucial component of LINQ, a powerful language feature that lets you query data from arrays, lists, databases, and XML.
- Functional programming support: Lambda expressions are an essential part of functional programming in C#. They let you write code in a more practical way, which can make the code stronger, easier to test, and easier to maintain.
- Event handling: Without having to define a separate named method, lambdas can provide a compact and simple way to define event handlers when handling events in C#.
- Asynchronous programming: Lambda Expression can be used to define callback functions or delegate expressions that are executed when a task completes in async/await programming.
Syntax of Lambda Expression
The syntax for a lambda function in C# is to use the lambda operator => and this separates the input parameter(s) from the expression representing the function’s body.
input => expression;
Types of Lambda Expression in C#
There are mainly 2 types of Lambda Expression in C#.
Expression Lambda – It contains a single expression within a lambda body.
For eg –
var square = (int n) => n * n;
Statement Lambda – The body of the statement lambda contains one or more statements. So, we use curly braces for the Lambda Statement. For example –
var sum = (int num1, int num2) =>
{
int result = num1 + num2;
return result;
};
Console.WriteLine("Sum = : " + sum(10, 8));
Examples of Lambda Expression with Code Snippet
Here’s an example of using a lambda expression in C#:
// Create a list of integers
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
// Use a lambda expression to filter the list
List<int> evenNumbers = numbers.Where(n => n % 2 == 0).ToList();
// Output the even numbers
foreach (int number in evenNumbers)
{
Console.WriteLine(number);
}
In this example, we begin by creating an integer list. Then Lambda Expression filters the list and returns only even numbers. The lambda expression n => n % 2 == 0
is used as a predicate to determine if each number is even or not. Finally, we use a for-each loop to print the even numbers to the console.
Here’s an example of using a lambda expression in C# to filter data based on a field.
Create a class and declare some property.
class Product
{
public string Name { get; set; }
public string Description { get; set; }
public string Category { get; set; }
}
Write a function which contains a List and then use Lambda expression to filter the data.
public void ProductInfo()
{
List<Product> details = new List<Product>() {
new Product { Name = "Laptop", Description = "New Laptop", Category = "Electronics" },
new Product { Name = "Desktop", Description = "Refutbished Laptop", Category = "Electronics" },
new Product { Name = "Keyboard", Description = "New Keyboard", Category = "Accessories" },
new Product { Name = "Mouse", Description = "New Mouse", Category = "Accessories" }
};
var itemDetails = details.OrderBy(x => x.Category == "Electroincs");
foreach (var prd in itemDetails)
{
Console.WriteLine(prd.Name + "--------------" + prd.Description );
}
}
Output –
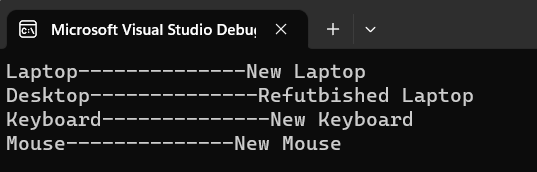
Lambda as Event Handler in C#
The below code snippet shows an event handler using Lambda.
public MainWindow()
{
InitializeComponent();
Loaded += (o, e) =>
{
this.Title = "Loaded";
};
}
Conclusion
Remember the below points about Lambda Expression in C#.
- Lambda is a shorter way to define the anonymous method.
- We can use multiple parameters in a Lambda Expression.
- Lambda can contain a single statement or multiple statements.
- We can use Lambda Expression in the same way as a delegate.
Hope you like this article. Please write your comment below and share this article within your tech group.
Keep following – SharePointCafe.Net