In this article, we will learn Prototype Pattern in detail and then we will see how to implement this pattern in C# .NET Core based application.

What is Prototype Pattern?
A prototype pattern is basically to copy the objects to save time and resources while creating new instances. This pattern is the part of Creational Pattern.
Developers use Prototype design pattern when the Object creation is an expensive undertaking and requires a ton of time and assets and you have a comparative item previously existing. Prototype pattern gives a component to duplicate the first item to another object and afterwards adjust it as per the requirements.
How Prototype Design Pattern Works?
The Prototype pattern designates the cloning system to the real objects that are being cloned. The pattern proclaims a typical interface for all objects that help to clone. This interface allows you to clone an object without coupling your code to the class of that object.
How to implement Prototype Pattern?
As we know that, the Prototype pattern allows us to copy objects in order to save the resource. So, in C# we must understand Shallow Copy and Deep Copy concept which actually enables us to achieve Prototype Design Pattern.
You may read this also - Source Code Management Tools
What is Shallow Copy?
Shallow Copy stores the references of objects to the first memory address. Shallow Copy reflects changes made to the new/duplicated object in the first object.
Below code, snippet explains Shallow Copy Concept
class Program
{
static void Main(string[] args)
{
Products product1 = new Products() { ProductId = 1, ProductName = "Laptop" };
Products product2 = product1;
Console.WriteLine(product1.ProductName);
Console.WriteLine(product2.ProductName);
product2.ProductName = "Desktop"; //Change Product Name in 2nd Object
Console.WriteLine(product1.ProductName);
Console.WriteLine(product2.ProductName);
Console.ReadKey();
}
}
Below is the output of the below code.
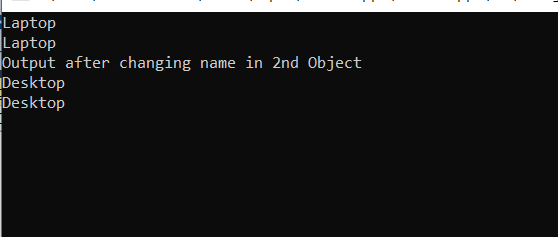
As we can see in the above code snippet, the second instance is being created as a copy from the first instance using equal(=)) operator. As a result, all the changes made in the first instance automatically reflects into the 2nd instance and vice-versa.
What is Deep Copy?
Deep Copy is an alternate of Shallow Copy in C#. In C#, Deep Copy alludes to a strategy by which a copy of an object is made with the end goal that it contains duplicates of both example individuals and the objects highlighted by reference individuals.
This means, Deep Copy allows the creation of actual copy but they do not share the same address in memory.
public class Products: ICloneable
{
public int ProductId { get; set; }
public string ProductName { get; set; }
public object Clone()
{
using (var ms = new MemoryStream())
{
var format = new BinaryFormatter();
format.Serialize(ms, this);
ms.Position = 0;
return (Products)format.Deserialize(ms);
}
}
}
The clone method will make sure that a new instance in the memory will be created. And any changes made in the original object will not reflect in the copy object because they are purely independent of each other.
Conclusion –
Prototype Design Pattern enables developers to create copies of objects in an efficient way in order to improve the performance of the system. We can use Shallow Copy or Deep Copy concept in C#. Shallow Copy maintains the same address in memory for all copied objects. Deep copy keeps distinct references in the memory.
Useful Article for you –
Source Code Management Tools Layered Architecture – Definitions and Uses Know everything about Good Software Architecture Evolution of .NET Framework to .NET Core